API category list
In Windows, a menu bar is a horizontal bar most often displayed immediately below the caption bar and usually containing a list of drop-down menu items. These drop-down menus can contain either other menu items or nested submenus. Menu items can be enabled, disabled, greyed and checked or not checked.
Menus can be constructed programmatically or built with a resource editor in some IDEs. CreateMenu(), AppendMenu() and InsertMenu() are the primary API function calls when building menus and maintaining menus at run time -
Create a Menu
To create a menu use the CreateMenu() API function. The menu is initially empty. The prototype of this function is
HMENU CreateMenu();
If the function succeeds, the return value is a handle to the newly created menu. If the function fails, the return value is NULL.
Adding a Menu Item
To add items to the top-level menu, drop-down menu, submenu or context menu use the AppendMenu API function. The prototype is -
BOOL AppendMenu(HMENU hMenu,UINT uFlags,UINT_PTR uIDNewItem,LPCSTR lpNewItem);
where
hMenu – A handle to the menu bar, drop-down menu, submenu, or shortcut menu to be changed.
uFlags -Controls the appearance and behaviour of the new menu item.
uIDNewItem – The identifier of the new menu item or, if the uFlags parameter is set to MF_POPUP, a handle to the drop-down menu or submenu.
lpNewItem -The content of the menu item. Can be one of the following: MF_BITMAP; MF_OWNERDRAW; MF_STRING
If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
For further detailed reading
https://docs.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-appendmenua
Dynamically Modifying Menus
Modify a Menu
The ModifyMenu function changes an existing menu item. The prototype of this function is -
BOOL ModifyMenu(HMENU hMnu,UINT uPosition,UINT uFlags,UINT uIDNewItem,LPCTSTR lpNewItem);
hMnu - Handle to the menu to be changed.
uPosition - Specifies the menu item to be changed, as determined by the uFlags parameter.
uFlags - Specifies flags that control the interpretation of the uPosition parameter and the content, appearance, and behaviour of the menu item.
uIDNewItem - Specifies either the identifier of the modified menu item or the handle to the drop-down menu or submenu.
lpNewItem - Pointer to the content of the changed menu item.
Returns nonzero if the function is successful; otherwise zero.
For further detailed reading
https://docs.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-modifymenua
Insert a Menu Item
To insert a new menu item at the specified position in a menu use the IntertMenuItem() API function. The prototype is -
BOOL InsertMenuItem(HMENU hmenu,UINT item,BOOL fByPosition,LPCMENUITEMINFOA lpmi);
hMenu - Handle to the menu where the new menu item will be inserted.
uItem - Identifier or position of the menu item before which to insert the new item.
fByPosition - Value specifying the meaning of uItem. If this parameter is FALSE, the uItem parameter is a menu item identifier. Otherwise, it is a menu item position.
lpmii - Pointer to a MENUITEMINFO structure that contains information about the new menu item.
Returns nonzero if the function is successful; otherwise zero.
For further detailed reading.
https://docs.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-insertmenuitema
Deleting a Menu
Deletes a menu item and the submenu associated with it, if any. The prototype of this function is -
BOOL DeleteMenu(HMENU hMenu,UINT uPosition, UINT uFlags);
Where
hMenu– Handle to the menu to be changed.
uPosition – Specifies the menu item that is to be deleted
nFlags – Is used to interpret nPosition
Returns nonzero if the function is successful; otherwise zero.
Some Useful Menu-Related Messages
WM_COMMAND – fires when the user selects an active menu item. The value of the LOWORD(wParam) contains the menu item selected.
WM_INITMENU – fires whenever the user clicks anywhere in the menu bar but before the menu becomes active allowing an application to change the menu before an item is chosen. Windows sends the WM_INITMENU message only once per menu activation. The wParam contains a handle to the menu to be initialised.
For further reading https://docs.microsoft.com/en-us/windows/win32/menurc/wm-initmenu
WM_MENUSELECT – Sent to a menu's owner window when the user selects a menu item. The low-order word of wParam specifies the menu item or submenu index while the high-order word of wParam specifies one or more menu flags. The lparam contains a handle to the menu that was clicked.
For further reading https://docs.microsoft.com/en-us/windows/win32/menurc/wm-menuselect
WM_INITMENUPOPUP – Sent when a drop-down menu or submenu is about to become active. This allows an application to modify the menu before it is displayed. The wParam is a handle to the drop-down menu or submenu. The low-order lParam word specifies the zero-based relative position of the menu item while the high-order word indicates whether the drop-down menu is the window menu. If the menu is the window menu, this parameter is TRUE; otherwise, it is FALSE.
For further reading https://docs.microsoft.com/en-us/windows/win32/menurc/wm-initmenupopup
The Popup or Context Menu
A popup or context menu generally appears when the user right-clicks. The term context menu refers to the fact that the options in the menu relate to what was right-clicked. The TrackPopupMenu() function displays a context menu in a specific position on the screen. The popup menu can be loaded from an existing resource or created dynamically with a call to CreatePopupMenu.
The prototype for the TrackPopupMenu is -
BOOL TrackPopupMenu(HMENU hMenu,UINT uFlags,int x,int y,int nReserved,HWND hWnd,const RECT *prcRect);
Where
nFlags – Specifies screen-position and mouse-position flags.
Use one of the following flags to specify how the function positions the shortcut menu horizontally.
TPM_CENTERALIGN – Centers the shortcut menu horizontally relative to the coordinate specified by the x parameter.
TPM_LEFTALIGN – Positions the shortcut menu so its left side is aligned with the coordinate specified by the x parameter.
TPM_RIGHTALIGN – Positions the shortcut menu so its right side is aligned with the coordinate specified by the x parameter.
Use one of the following flags to specify how the function positions the shortcut menu vertically.
TPM_BOTTOMALIGN – Positions the shortcut menu so its bottom side is aligned with the coordinate specified by the y parameter.
TPM_TOPALIGN – Positions the shortcut menu so its top side is aligned with the coordinate specified by the y parameter.
TPM_VCENTERALIGN – Centers the shortcut menu vertically relative to the coordinate specified by the y parameter.
x – Specifies the horizontal position in screen coordinates of the pop-up menu.
y – Specifies the vertical position in screen coordinates of the top of the menu on the screen.
pWnd – Identifies the window that owns the pop-up menu.
lpRect – Ignored.
Returns the result of calling TrackPopupMenu
Example
- Details
- Category: API category list
- Hits: 117
A child window or control is a prepacked window that exists inside and depends on a parent window. Controls reduce repetitive development tasks and promote a consistent and recognisable application interface. The child window processes mouse and keyboard messages and notifies the parent window, by way of command messages when the child window’s state has changed. Child controls are created using the CreatWindowEx() or CreateWindow() function using a predefined class parameter in the CreateWindow call. Windows offers more than 20 types of controls; six, are known as classic controls and are implemented as standard.
Edit Control
An edit or textbox control is used to enter and edit text. The text in the edit controls can be left-justified, right-justified, or centred. Edit controls default to a single line but can be created as multiline. An edit control is limited to about 60 KB of text. If there is a requirement to handle large amounts of text, an enhanced version of the standard edit control known as the rich edit control is available as part of the common control library. The predefined class name for a textbox in the CreatWindow function is "EDIT"
Example
In the following, the user can enter a limited amount of text into the edit box. Clicking the button title 'set title' copies Editbox contents to the static box.
Static Control
Static controls are commonly used as labels for other controls. The static control cannot be selected, accept input from the keyboard or mouse, and does not send WM_COMMAND messages back to the parent window. A static control can display text, shapes, icons or bitmaps. A static window is created using “STATIC” as the window class in the CreateWindow function. For an overview of its configuration and use, see the editbox example (above).
Buttons
A Button is a simple control used to trigger an action. When a button is clicked, it sends a WM_COMMAND message to the parent window.
Push Button
A push or command button is a rectangular clickable window typically containing a text label describing its function. The predefined class name used in the CreateWindow function is "BUTTON". For an overview of its configuration and use, see the editbox example (above).
Check Boxes
A checkbox is a small square box and associated label that describes the purpose of the checkbox. Checkboxes function as a toggle switch allowing the selection and de-selection options. Clicking the box once causes a checkmark to appear; clicking it again toggles the checkmark off. Both of these actions send a message to the parent window. The predefined class name used for a checkbox in the Creatwindow function is "BUTTON" with the dwStyle being set to "BS_CHECKBOX".
Example
In the example below, clicking any checkboxes changes the window's background to a mixture of the corresponding checkbox colour.
Radio Buttons
A radio button allows the user to choose only one of a predefined set of mutually exclusive options. The control consists of a small circle and a descriptive label. Radio buttons are arranged in groups of two or more and are mutually exclusive. When the user selects a particular radio button, any previously selected button in the same group becomes deselected. Unlike checkboxes, radio buttons do not work as toggles. The predefined class name for a radio button in the Creatwindow function is "BUTTON" with the dwStyle being set to "BS_AUTORADIOBUTTON"
Example
In the example below, clicking any radiobutton changes the window's background to the corresponding radiobutton colour.
The Scrollbar
A scroll bar is a control that allows the user to adjust a particular value or a section of the window or view, by navigating either left and right or up and down. A scroll bar appears as a long bar with a small button at each end. Between these buttons, there is a moveable bar called a thumb. Scrollbars can either be integral to the window or be a separate control.
The predefined class for the scrollbar is SCROLLBAR. To add a scrollbar to an existing window add the WS_HSCROLL and WS_VSCROLL parameters to the dwStyle parameter when creating the window.
Example
The following short program demonstrates the main window's vertical scrollbar by displaying the scroll value within the main window.
The Listbox Control
A listbox displays a list of items displayed as a scrollable list within a rectangle. Users can select or deselect one or more of these items by clicking the appropriate line of text. The predefined class for the listbox is “LISTBOX”
Example
The following short program creates a list box and then adds a limited number of selectable items. Clicking any list item results in the selected listview value being copied to the static box.
A Combo Box Control
A combo box or drop-down list is a combination of a listbox and editbox, allowing the user to either type a value directly or select a value from the list. Combo boxes come in three varieties: simple, drop-down, and drop-down list. The predefined class for the scrollbar is “COMBOBOX”
Example
The following short program creates a dropdown list and then adds a limited number of selectable items. Selecting any list item results in the selected value being copied to the static box.
- Details
- Category: API category list
- Hits: 121
A dialog box is a temporary popup window that prompts the user for additional information. A dialog box will usually contain one or more controls (child windows) with which the user can enter text, choose options, or direct the action of the application.
In addition to user-defined dialogue boxes, Windows also provides predefined dialog boxes that support common menu items such as colour selection and an open file interface.
Modal v Modeless Dialogue Box
Dialog boxes can be either modeless or modal. A modal dialogue box will not allow the user to switch between the dialog box and any other window without explicitly closing the dialog box. A modeless dialog box does not prevent the user from switching to other parts of the application.
Creating a Modeless Dialog Box
Modeless dialog boxes are created using the API function CreateDialog(). The syntax is below
hDlgModeless = CreateDialog (hInstance, lpTemplate, hWndParent, lpDialogFunc) ;
hInstance - A handle to the current application.
lpTemplate - specifies the resource identifier of the dialog box template.
hWndParent - A handle to the parent window that owns the dialog box.
lpDialogFunc - A pointer to the dialog box procedure.
Return value - If the function succeeds, the return value is the dialog box handle. If the function fails, the return value is NULL.
Deactivating a Modeless Dialog Box
To destroy the modeless dialog box, use the DestroyWindow() function.
BOOL DestroyWindow(HWND hWnd);
Where HWnd is a handle to the window to be destroyed. If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
Creating a Modal Dialog Box
Modal dialog boxes are created using the APO function DialogBox(). The syntax is
void DialogBox(hInstance,lpTemplate,hWndParent,lpDialogFunc);
hInstance - A handle to the current application.
lpTemplate - Specifies the resource identifier of the dialog box template.
hWndParent - A handle to the parent window that owns the dialog box.
lpDialogFunc - A pointer to the dialog box procedure.
Deactivating a Modal Dialog Box
To deactivate and close a modal dialogue box use the EndDialog() API function call. The syntax of this function is
BOOL EndDialog(HWND hDlg,INT_PTR nResult);
HDlg - A handle to the dialog box to be destroyed.
nResult - The value to be returned to the application from the function that created the dialog box.
Return value - If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
Processing Dialog Messages
Each dialogue box will have its version of the window call-back function (wndproc) and receive messages in the same fashion as the main window. The dialog callback function, however, differs from its main Windows counterpart in that it returns a boolean value.
Example
The code example demonstrates a modal and modeless dialog box. The modal dialog box displays a simple message. The modeless dialog box has 3 radio buttons. Selecting any of the radiobutton changes the background colour of the parent window.
Dialog-Based Applications
Creating a program where the main window is a dialog box is referred to as a dialog-based application. This method simplifies the application build process by making it easier to place controls onto the main window using the system resource dialog editor. A dialog box procedure can then be added to react to events that occur in the dialog box. Dialog-based applications are much simpler, architectually, than other designs therefor dialogs appliation are best suited for creating rudimentary apps.
The following example creates a simple dialog-based application. The dialog box title can be changed to the value in the textbox by clicking the button control.
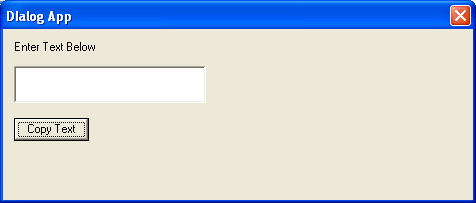
- Details
- Category: API category list
- Hits: 145
The Common Dialog Box Library consists of a collection of functions for invoking standard dialog boxes for performing common tasks. There are standard Windows dialogs for opening and saving files, choosing a printer, finding and replacing text, setting font options, picking a colour, and using a help file. Using the common control dialog enables an application to maintain a look of consistency. The common dialog box library files are found in COMMDLG.H header file. To use these functions, the application must first initialise the fields of a structure and then pass a structure pointer to the function. When the user terminates the dialog box, control returns to the calling application with any relevant information passed back to the application via the dialog box structure.
For further reading https://docs.microsoft.com/en-us/windows/win32/dlgbox/dialog-box-types
The examples below demonstrate 3 common dialog boxes: text find and replace, the file open and save and colour select.
Select Colour Common Dialog Box
The Select Colour Dialog Box displays a basic set of available colours in addition to allowing a user to create custom colours by specifying RGB values
File Open/Save Common Dialog Box
The ‘Common File-Open/Save’ dialog offers a consistent way to deal with file management operations, using the standard dialog interface that Windows users should be familiar with.
The Open dialog box lets the user specify the drive, directory, and the name of a file or set of files to open.
The Save As dialog box lets the user specify the drive, directory, and name of a file to save.
Find Common Dialog Box
The Find and Replace Common Dialog Box displays a modeless dialog box that allows the user to specify a string to search for within a text document, as well as additional options for text editing
- Details
- Category: API category list
- Hits: 134
Windows supports two types of bitmap: device-independent (DIB) and device-dependent(DDB). A device-independent bitmap is an external format, which allows bitmaps to be moved from one device to another. Device-dependent bitmaps are designed and optimised for use with a specific device (device-dependent) and hence are unsuitable for use in an environment for which they were not created. A typical example would be a bitmap created for video memory optimised for displaying screen output.
Creating and Loading Device-Independent Bitmaps
Device-independent bitmaps are typically created using an image editor. To load a bitmap for use in an application use the LoadImage() function. This supersedes the LoadBitmap() function. The prototype for this function is
HANDLE LoadImage(HINSTANCE hInst, LPCSTR name, UINT type, int cx, int cy, UINT fuLoad);
Where
hInst - is a handle to the module of either a DLL or executable (.exe) that contains the image to be loaded.
name - the image to be loaded.
UINT - The type of image to be loaded.
cx - The width, in pixels, of the icon or cursor.
cy- The height, in pixels, of the icon or cursor.
fuLoad - can be one of the following values: LR_CREATEDIBSECTION; LR_DEFAULTCOLOR;LR_DEFAULTSIZE; LR_LOADFROMFILE; LR_LOADMAP3DCOLORS; LR_LOADTRANSPARENT; LR_MONOCHROME; LR_SHARED; LR_VGACOLOR
If the function succeeds, the return value is the handle of the newly loaded image. If the function fails, the return value is NULL.
For further reading
https://docs.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-loadimagea
To convert a DIB to a DDB, use the API function CreateDIBitmap.
https://docs.microsoft.com/en-us/windows/win32/api/wingdi/nf-wingdi-createdibitmap
Displaying Bitmaps
To display a bitmap, two device contexts must be declared: one will hold the current device context while the other will create a second copy device context that will be used to store the bitmap until it is ready to be drawn in the window. This copy device context is created using a call to API function CreatCompatibleDC(). A newly created or existing bitmap can then be selected into the copy device context using the SelectObject() API function and finally copied to the screen using the BitBlt() API function. The sequence of events is as follows -
DC=GetDC(hwnd); memDC=CreateCompatibleDC(DC); SelectObject(memDC,bitmap1); BitBlt(DC,x.y,cx,cy, memdc,x1,y1,SRCCOPY);
The syntax for the CreateCompatibleDC function is
HDC CreateCompatibleDC(HDC hdc);
Where hdc is a handle to an existing DC. If this handle is NULL, the function creates a memory DC compatible with the application's current screen. If the function succeeds, the return value is the handle to a memory DC. If the function fails, the return value is NULL.
The syntax for this Bitblt() copy function is
BOOL BitBlt(HDC hdc,int x,int y,int cx, int cy,HDC hdcSrc,int x1,int y1,DWORD rop);
where
hdc - handle to the destination device context.
x - The x-coordinate upper-left corner of the destination rectangle.
y - The y-coordinate of the upper-left corner of the destination rectangle.
cx - The width of the source and destination rectangles.
cy - The height, in logical units, of the source and the destination rectangles.
HdcSrc - A handle to the source device context.
x1 - The x-coordinate of the upper-left corner of the source rectangle.
y1 - The y-coordinate of the upper-left corner of the source rectangle.
rop - A raster-operation code. Define how the colour data for the source rectangle will be combined with the destination rectangle. The 'vanilla' operating code SRCCOPY will copy the source rectangle directly onto the destination rectangle. For additional raster code information see the link below
Bltbmp returns zero if successful and non-zero otherwise.
When a bitmap is no longer needed it must be destroyed using the DeleteObject() API function.
For further information
https://docs.microsoft.com/en-us/windows/win32/api/wingdi/nf-wingdi-bitblt
Repainting the Screen Using Device Dependent Bitmaps
Using a bitmap to create a copy of the device context offers one solution to the problem of saving the contents of the client area before a repaint request. All screen output is directed to this virtual window and each time a repaint is received, the contents of the virtual window are then copied to the screen's device context.
Creating Device-Dependent Bitmaps
The API function CreateCompatibleBitmap() creates a bitmap compatible with the current device context handle. This function is best used for creating colour bitmaps. The prototype of this API function is
HBITMAP CreateCompatibleBitmap (hdc, cx, cy) ;
Where
hdc is a handle to a device context
cx is the bitmap width, in pixels
cy is the bitmap height, in pixels.
If the function succeeds, the return value is a handle to the compatible bitmap (DDB). If the function fails, the return value is NULL.
In addition, a Windows application can also create a device-dependent bit using the CreateBitmap API function. This function is best used for creating monochrome bitmaps. The syntax for this function is
HBITMAP CreateBitmap(int nWidth,int nHeight,UINT nPlanes,UINT nBitCount,const VOID *lpBits);
where
nWidth - The bitmap width, in pixels.
nHeight - The bitmap height, in pixels.
nPlanes - The number of colour planes used by the device.
nBitCount - The number of bits required to identify the colour of a single pixel.
lpBits - Set the colours in a rectangle of pixels.
If the function succeeds, the return value is a handle to a bitmap. If the function fails, the return value is NULL.
Example
In the code section below, Windows creates a device context of the application window and a compatible device context. The application then creates and fills this virtual copy with 200 random lines. Each time a repaint request is received, the contents of the virtual window are copied to the screen device context by the BitBlt function.
Copying a Bitmap Using the StretchBlt Function
The StretchBlt() function copies a bitmap from a source rectangle into a destination rectangle, stretching or compressing the bitmap to fit the dimensions of the destination rectangle. In the code section below Windows creates a bitmap of the existing screen display and then copies it to the application window using StretchBlt API function. Clicking the left mouse button anywhere with the client Window will initiate the screen copy action.
Example
In the code section below Windows creates a bitmap of the existing screen display and then copies it to the application window using StretchBlt API function. Clicking the left mouse button anywhere with the client Window will initiate the screen copy action.
- Details
- Category: API category list
- Hits: 107